45 how to do label encoding in python for multiple columns
python 3.x - How to encode multiple categorical columns for test data ... colnames= [' {}_ {}'.format (col,val) for col,unique_values in zip (df.columns,ohe.categories_) \ for val in unique_values] pd.DataFrame (ohe.fit_transform (df), columns=colnames) Update: If you are fine with ordinal endocing, the following change could help. How to Perform One-Hot Encoding in Python - Statology Step 2: Perform One-Hot Encoding. Next, let's import the OneHotEncoder () function from the sklearn library and use it to perform one-hot encoding on the 'team' variable in the pandas DataFrame: Notice that three new columns were added to the DataFrame since the original 'team' column contained three unique values. Note: You can find ...
How to do Label Encoding across multiple columns - Kaggle There are multiple ways to do it. I usually follow below method: Let me know if you need more info around this. P.S: I'm sure we are not confused between Label Encoding and One Hot. If we are, below code should do for One Hot encoding: pd.get_dummies(df,drop_first=True) HTH
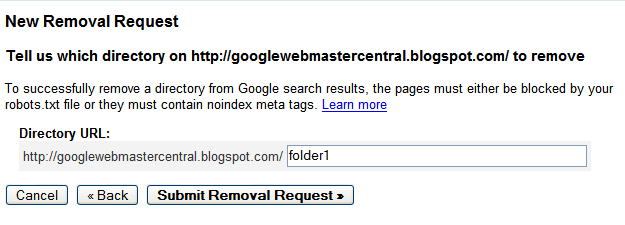
How to do label encoding in python for multiple columns
Encoding Categorical Values, Python- Scikit-Learn - Medium In Data science, we work with datasets which has multiple labels in one or more columns. They can be in numerical or text format of any encoding. This will be ideal and understandable by humans. LabelEncoder Example - Single & Multiple Columns - Data Analytics # Encode labels of multiple columns at once # df [cols] = df [cols].apply (LabelEncoder ().fit_transform) # # Print head # df.head () This is what gets printed. Make a note of how columns related to workex, status, hsc_s, degree_t got encoded with numerical / integer value. Fig 4. Multiple columns encoded with integer values using LabelEncoder How to use label encoding through Python on multiple ... - ResearchGate to find what kind of encoding should be used to handle such categorical data. If we consider hierarchical clustering algorithms, they use distances between objects/items to be clustered and form...
How to do label encoding in python for multiple columns. Encode and decode a column of a dataframe in python - pandas In this tutorial we will learn how to encode and decode a column of a dataframe in python pandas. We will see an example to encode a column of a dataframe in python pandas and another example to decode the encoded column. Encode a column of dataframe in python: Create dataframe: ML | Label Encoding of datasets in Python - GeeksforGeeks We apply Label Encoding on iris dataset on the target column which is Species. It contains three species Iris-setosa, Iris-versicolor, Iris-virginica . Python3 import numpy as np import pandas as pd df = pd.read_csv ('../../data/Iris.csv') df ['species'].unique () Output: array ( ['Iris-setosa', 'Iris-versicolor', 'Iris-virginica'], dtype=object) Categorical encoding using Label-Encoding and One-Hot-Encoder Label Encoding This approach is very simple and it involves converting each value in a column to a number. Consider a dataset of bridges having a column names bridge-types having below values. Though there will be many more columns in the dataset, to understand label-encoding, we will focus on one categorical column only. BRIDGE-TYPE Arch Beam Comparing Label Encoding And One-Hot Encoding With Python Implementation In Python, label encoding can be done with the help of the Sklearn library. We used label encoder for specifically two columns or class which are "sex" and "embarked". After appling label encoder we can notice that in embarked class C, Q and S are assumed as 0,1 and 2 respectively while the male and female in sex class is assumed as 1 ...
How to reverse Label Encoder from sklearn for multiple columns ... - Python This is the code I use for more than one columns when applying LabelEncoder on a dataframe: 25. 1. class MultiColumnLabelEncoder: 2. def __init__(self,columns = None): 3. self.columns = columns # array of column names to encode. 4. Label Encoding in Python - A Quick Guide! - AskPython Python sklearn library provides us with a pre-defined function to carry out Label Encoding on the dataset. Syntax: from sklearn import preprocessing object = preprocessing.LabelEncoder () Here, we create an object of the LabelEncoder class and then utilize the object for applying label encoding on the data. 1. Label Encoding with sklearn Label Encoding in Python - Machine Learning - PyShark Label Encoding in Python In this part we will cover a few different ways of how to do label encoding in Python. Two of the most popular approaches: LabelEncoder () from scikit-learn library pandas.factorize () from pandas library Once the libraries are downloaded and installed, we can proceed with Python code implementation. python - Label encoding across multiple columns in scikit-learn - Stack ... A short way to LabelEncoder () multiple columns with a dict () : from sklearn.preprocessing import LabelEncoder le_dict = {col: LabelEncoder () for col in columns } for col in columns: le_dict [col].fit_transform (df [col]) and you can use this le_dict to labelEncode any other column:
How to perform one hot encoding on multiple categorical columns If you really need to do it this way: categorical_cols = ['a', 'b', 'c', 'd'] from sklearn.preprocessing import LabelEncoder # instantiate labelencoder object le = LabelEncoder () # apply le on categorical feature columns data [categorical_cols] = data [categorical_cols].apply (lambda col: le.fit_transform (col)) from sklearn.preprocessing import ... Label Encoding in Python - Javatpoint Explanation: In the above example, we have imported pandas and preprocessing modules of the scikit-learn library. We have then defined the data as a dictionary and printed a data frame for reference. Later on, we have used the fit_transform() method in order to add label encoder functionality pointed by the object to the data variable. We have printed the unique code with respect to the Gender ... Ordinal Encoding in Python - KoalaTea Using a Label Encoder in Python. To encode our cities, turn them into numbers, we will use the OrdinalEncoder class from the category_encoders package. We first create an instance of the class. We need to pass the cols it will encode cols = ['shirts'] and we can also pass a mapping which will tell the encoder the order of our categories. The mapping is optional, but allows us to control the order. One hot Encoding with multiple labels in Python? - DeZyre Step 1 - Import the library Step 2 - Setting up the Data Step 3 - Using MultiLabelBinarizer and Printing Output Step 1 - Import the library from sklearn.preprocessing import MultiLabelBinarizer We have only imported MultiLabelBinarizer which is reqired to do so. Step 2 - Setting up the Data
How to Encode Categorical Columns Using Python - Medium First, we will reformat columns with two distinct values. They are the ever_married and the residence_type column. For doing that, we can use the LabelEncoder object from scikit-learn to encode the columns. Now let's take the ever_married column. First, we will initialize the LabelEncoder object like this:
Categorical Data Encoding with Sklearn LabelEncoder and OneHotEncoder Apply Sklearn Label Encoding The Sklearn Preprocessing has the module LabelEncoder () that can be used for doing label encoding. Here we first create an instance of LabelEncoder () and then apply fit_transform by passing the state column of the dataframe. In the output, we can see that the values in the state are encoded with 0,1, and 2. In [3]:
Label Encoding on multiple columns | Data Science and Machine ... - Kaggle Create a list from your dataframe columns (3 columns in your dataset) label_object ['Product'].inverse_transform (df ['Product']) You can use the below code on your data frame, it label encoding will be applied on all column. You can use df.apply () to apply le.fit_transform to multiple columns:
Label encoding across multiple columns in scikit-learn. Learn Python at ... The classically Pythonic way, available in Python 2 and Python 3.0-3.4, is to do this as a two-step process: z = x.copy() z.update(y) # which returns None since it mutates z In both approaches, y will come second and its values will replace x "s values, thus b will point to 3 in our final result. Not yet on Python 3.5, but want a single expression
ML | One Hot Encoding to treat Categorical data parameters One-Hot encoding the categorical parameters using get_dummies () Python3 one_hot_encoded_data = pd.get_dummies (data, columns = ['Remarks', 'Gender']) print(one_hot_encoded_data) Output: We can observe that we have 3 Remarks and 2 Gender columns in the data. However, you can just use n-1 columns to define parameters if it has n unique labels.
Categorical Encoding with Pandas: get_dummies - Medium The "country" column has 4 unique values, which means we will get 4 columns after applying get_dummies (). With this syntax we can apply get_dummies to a column of dataframe; static = pd.get ...
Label encode multiple columns for training and scoring dataframes import pandas as pd from sklearn.preprocessing import LabelEncoder #Create the function to fit and transform the label encoder def label_encode_columns(df, columns): encoders = {} for col in columns: le = LabelEncoder().fit(df[col]) df[col] = le.transform(df[col]) encoders[col] = le return df, encoders #Create the function to take in the fitted encoders and transform the scoring dataset def label_encode_columns_w_fit_encoders(df, columns, encoders): for col in columns: le = encoders.get(col ...
Guide to Encoding Categorical Values in Python - Practical Business Python Label encoding is simply converting each value in a column to a number. For example, the body_style column contains 5 different values. We could choose to encode it like this: convertible -> 0 hardtop -> 1 hatchback -> 2 sedan -> 3 wagon -> 4 This process reminds me of Ralphie using his secret decoder ring in "A Christmas Story"
Post a Comment for "45 how to do label encoding in python for multiple columns"